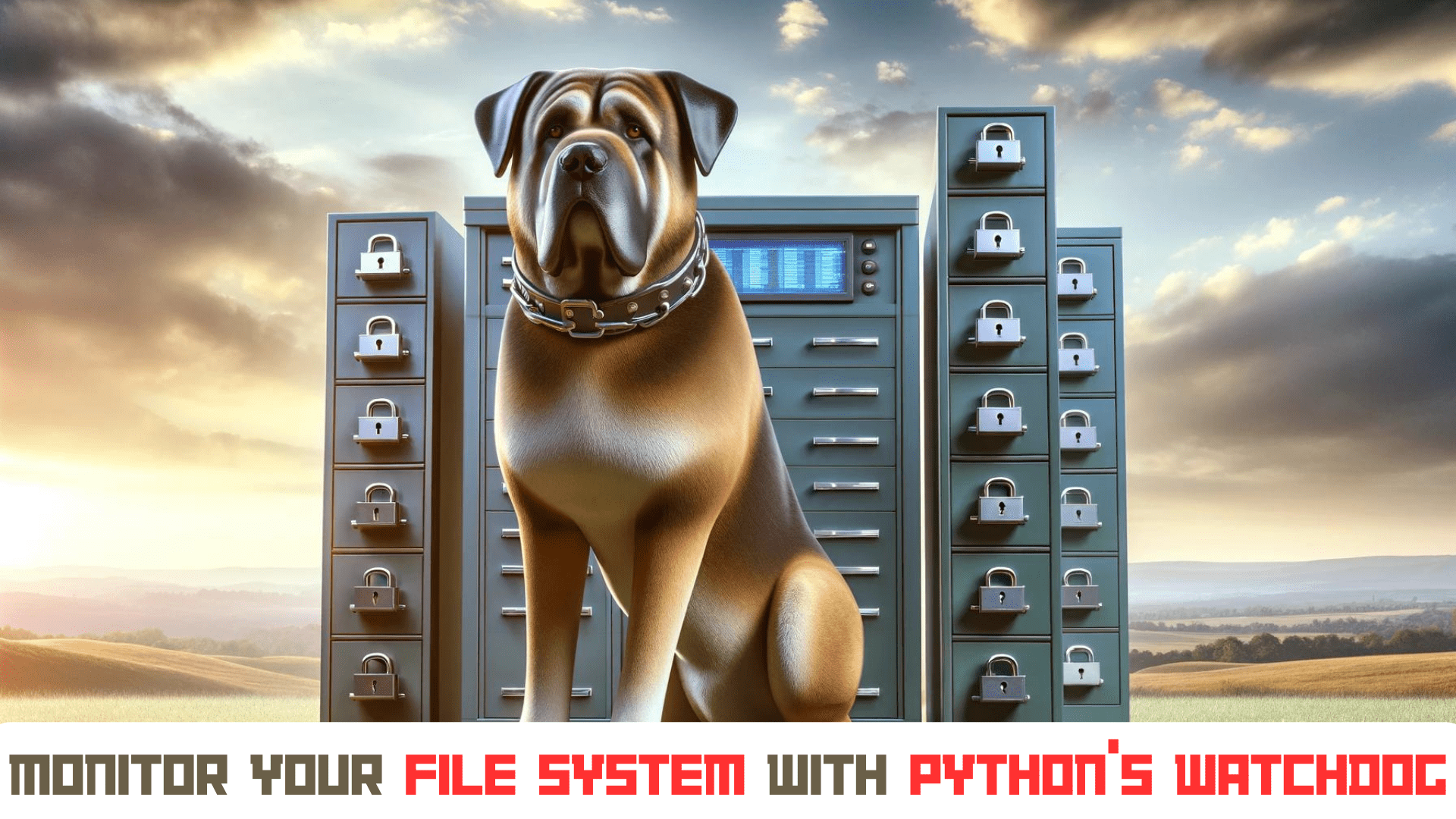
Picture by Creator | DALLE-3 & Canva
Python’s watchdog library makes it straightforward to watch your file system and reply to those adjustments routinely. Watchdog is a cross-platform API that lets you run instructions in response to any adjustments within the file system being monitored. We are able to set triggers on a number of occasions reminiscent of file creation, modification, deletion, and motion, after which reply to those adjustments with our customized scripts.
Setup for Watchdog
You may want two modules to start:
- Watchdog: Run this command beneath within the terminal to put in the watchdog.
- Logging: It’s a built-in Python module, so there isn’t a have to externally set up it.
Primary Utilization
Let’s create a easy script ‘foremost.py’ that screens a listing and prints a message every time a file is created, modified, or deleted.
Step 1: Import Required Modules
First, import the mandatory modules from the watchdog library:
import time
from watchdog.observers import Observer
from watchdog.occasions import FileSystemEventHandler
Step 2: Outline Occasion Handler Class
We outline a category MyHandler that inherits from FileSystemEventHandler. This class overrides strategies like on_modified, on_created, and on_deleted to specify what to do when these occasions happen. The occasion handler object might be notified when any adjustments occur within the file system.
class MyHandler(FileSystemEventHandler):
def on_modified(self, occasion):
print(f'File {occasion.src_path} has been modified')
def on_created(self, occasion):
print(f'File {occasion.src_path} has been created')
def on_deleted(self, occasion):
print(f'File {occasion.src_path} has been deleted')
Some helpful strategies of FileSystemEventHandler
are defined beneath.
- on_any_event: Executed for any occasion.
- on_created: Executed upon creation of a brand new file or listing.
- on_modified: Executed upon modification of a file or when a listing is renamed.
- on_deleted: Triggered upon the deletion of a file or listing.
- on_moved: Triggered when a file or listing is relocated.
Step 3: Initialize and Run the Observer
The Observer class is accountable for monitoring the file system for any adjustments and subsequently notifying the occasion handler. It repeatedly tracks file system actions to detect any updates.
if __name__ == "__main__":
event_handler = MyHandler()
observer = Observer()
observer.schedule(event_handler, path=".", recursive=True)
observer.begin()
attempt:
whereas True:
time.sleep(1)
besides KeyboardInterrupt:
observer.cease()
observer.be a part of()
We begin the observer and use a loop to maintain it operating. While you need to cease it, you may interrupt with a keyboard sign (Ctrl+C)
.
Step 4: Run the Script
Lastly, run the script with the next command.
Output:
File .File1.txt has been modified
File .New Textual content Doc (2).txt has been created
File .New Textual content Doc (2).txt has been deleted
File .New Textual content Doc.txt has been deleted
The above code will log all of the adjustments within the listing to the terminal if any file/folder is created, modified, or deleted.
Superior Utilization
Within the following instance, we’ll discover how you can arrange a system that detects any change in Python information and run exams for it routinely. We have to set up pytest with the next command.
Step 1: Create a Easy Python Undertaking With Assessments
First, arrange the fundamental construction of your mission:
my_project/
│
├── src/
│ ├── __init__.py
│ └── instance.py
│
├── exams/
│ ├── __init__.py
│ └── test_example.py
│
└── watchdog_test_runner.py
Step 2: Write Code in Instance Python File
Create a easy Python module in src/instance.py:
def add(a, b):
return a + b
def subtract(a, b):
return a - b
Step 3: Write the Check Instances
Subsequent, write the check circumstances for capabilities in exams/test_example.py:
import pytest
from src.instance import add, subtract
def test_add():
assert add(1, 2) == 3
assert add(-1, 1) == 0
assert add(-1, -1) == -2
def test_subtract():
assert subtract(2, 1) == 1
assert subtract(1, 1) == 0
assert subtract(1, -1) == 2
Step 4: Write the Watchdog Script
Now, create the watchdog_test_runner.py script to watch adjustments in Python information and routinely run exams:
import time
import subprocess
from watchdog.observers import Observer
from watchdog.occasions import FileSystemEventHandler
class TestRunnerHandler(FileSystemEventHandler):
def on_modified(self, occasion):
if occasion.src_path.endswith('.py'):
self.run_tests()
def run_tests(self):
attempt:
end result = subprocess.run(['pytest'], verify=False, capture_output=True, textual content=True)
print(end result.stdout)
print(end result.stderr)
if end result.returncode == 0:
print("Assessments handed efficiently.")
else:
print("Some exams failed.")
besides subprocess.CalledProcessError as e:
print(f"Error operating exams: {e}")
if __name__ == "__main__":
path = "." # Listing to observe
event_handler = TestRunnerHandler()
observer = Observer()
observer.schedule(event_handler, path, recursive=True)
observer.begin()
print(f"Looking forward to adjustments in {path}...")
attempt:
whereas True:
time.sleep(1)
besides KeyboardInterrupt:
observer.cease()
observer.be a part of()
Step 5: Run the Watchdog Script
In the long run, open a terminal, navigate to your mission listing (my_project), and run the watchdog script:
python watchdog_test_runner.py
Output:
Looking forward to adjustments in ....
========================= check session begins =============================
platform win32 -- Python 3.9.13, pytest-8.2.1, pluggy-1.5.0
rootdir: F:Net Devwatchdog
plugins: anyio-3.7.1
collected 2 gadgets
teststest_example.py .. [100%]
========================== 2 handed in 0.04s ==============================
Assessments handed efficiently.
This output exhibits that every one the check circumstances are handed after adjustments have been made to instance.py file.
Summing Up
Python’s watchdog library is a strong device for monitoring your file system. Whether or not you are automating duties, syncing information, or constructing extra responsive purposes, watchdog makes it straightforward to react to file system adjustments in actual time. With only a few traces of code, you can begin monitoring directories and dealing with occasions to streamline your workflow.
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for knowledge science and the intersection of AI with drugs. She co-authored the book “Maximizing Productiveness with ChatGPT”. As a Google Era Scholar 2022 for APAC, she champions range and educational excellence. She’s additionally acknowledged as a Teradata Range in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower ladies in STEM fields.