[ad_1]
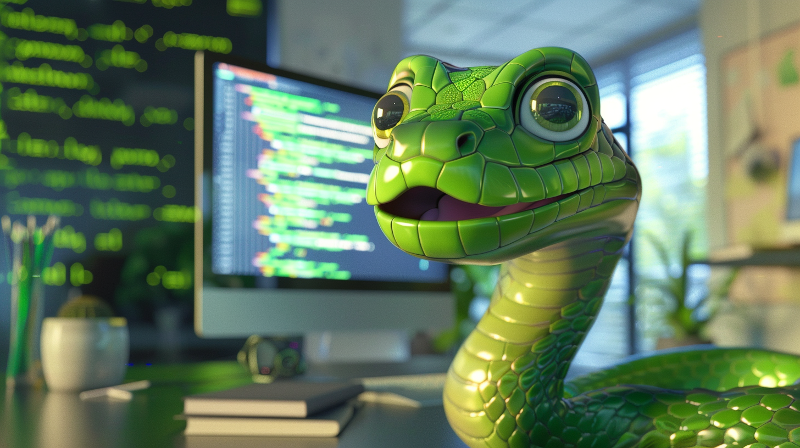
Picture generated with Midjourney
As an information skilled, it’s important to know find out how to course of your knowledge. Within the trendy period, it means utilizing programming language to rapidly manipulate our knowledge set to attain our anticipated outcomes.
Python is the most well-liked programming language knowledge professionals use, and lots of libraries are useful for knowledge manipulation. From a easy vector to parallelization, every use case has a library that might assist.
So, what are these Python libraries which might be important for Knowledge Manipulation? Let’s get into it.
1.NumPy
The primary library we might talk about is NumPy. NumPy is an open-source library for scientific computing exercise. It was developed in 2005 and has been utilized in many knowledge science instances.
NumPy is a well-liked library, offering many helpful options in scientific computing actions akin to array objects, vector operations, and mathematical features. Additionally, many knowledge science use instances depend on a posh desk and matrices calculation, so NumPy permits customers to simplify the calculation course of.
Let’s attempt NumPy with Python. Many knowledge science platforms, akin to Anaconda, have Numpy put in by default. However you may all the time set up them by way of Pip.
After the set up, we might create a easy array and carry out array operations.
import numpy as np
a = np.array([1, 2, 3])
b = np.array([4, 5, 6])
c = a + b
print(c)
Output: [5 7 9]
We will additionally carry out primary statistics calculations with NumPy.
knowledge = np.array([1, 2, 3, 4, 5, 6, 7])
imply = np.imply(knowledge)
median = np.median(knowledge)
std_dev = np.std(knowledge)
print(f"The info imply:{imply}, median:{median} and customary deviation: {std_dev}")
The info imply:4.0, median:4.0, and customary deviation: 2.0
It’s additionally potential to carry out linear algebra operations akin to matrix calculation.
x = np.array([[1, 2], [3, 4]])
y = np.array([[5, 6], [7, 8]])
dot_product = np.dot(x, y)
print(dot_product)
Output:
[[19 22]
[43 50]]
There are such a lot of advantages you are able to do utilizing NumPy. From dealing with knowledge to complicated calculations, it’s no marvel many libraries have NumPy as their base.
2. Pandas
Pandas is the most well-liked knowledge manipulation Python library for knowledge professionals. I’m certain that lots of the knowledge science studying lessons would use Pandas as their foundation for any subsequent course of.
Pandas are well-known as a result of they’ve intuitive APIs but are versatile, so many knowledge manipulation issues can simply solved utilizing the Pandas library. Pandas permits the consumer to carry out knowledge operations and analyze knowledge from numerous enter codecs akin to CSV, Excel, SQL databases, or JSON.
Pandas are constructed on high of NumPy, so NumPy object properties nonetheless apply to any Pandas object.
Let’s attempt on the library. Like NumPy, it’s normally out there by default in case you are utilizing a Knowledge Science platform akin to Anaconda. Nevertheless, you may comply with the Pandas Set up information in case you are not sure.
You may attempt to provoke the dataset from the NumPy object and get a DataFrame object (Desk-like) that exhibits the highest 5 rows of information with the next code.
import numpy as np
import pandas as pd
np.random.seed(0)
months = pd.date_range(begin="2023-01-01", intervals=12, freq='M')
gross sales = np.random.randint(10000, 50000, measurement=12)
transactions = np.random.randint(50, 200, measurement=12)
knowledge = {
'Month': months,
'Gross sales': gross sales,
'Transactions': transactions
}
df = pd.DataFrame(knowledge)
df.head()


df[df['Transactions'] <100]
It’s potential to do the Knowledge calculation.
total_sales = df['Sales'].sum()
average_transactions = df['Transactions'].imply()
Performing knowledge cleansing with Pandas can also be simple.
df = df.dropna()
df = df.fillna(df.imply())
There may be a lot to do with Pandas for Knowledge Manipulation. Try Bala Priya article on utilizing Pandas for Knowledge Manipulation to be taught additional.
3. Polars
Polars is a comparatively new knowledge manipulation Python library designed for the swift evaluation of enormous datasets. Polars boast 30x efficiency positive factors in comparison with Pandas in a number of benchmark assessments.
Polars is constructed on high of the Apache Arrow, so it’s environment friendly for reminiscence administration of the massive dataset and permits for parallel processing. It additionally optimize their knowledge manipulation efficiency utilizing lazy execution that delays and computational till it’s needed.
For the Polars set up, you need to use the next code.
Like Pandas, you may provoke the Polars DataFrame with the next code.
import numpy as np
import polars as pl
np.random.seed(0)
employee_ids = np.arange(1, 101)
ages = np.random.randint(20, 60, measurement=100)
salaries = np.random.randint(30000, 100000, measurement=100)
df = pl.DataFrame({
'EmployeeID': employee_ids,
'Age': ages,
'Wage': salaries
})
df.head()
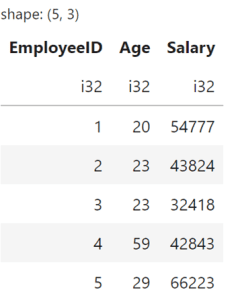
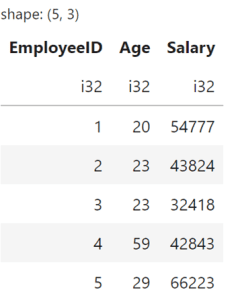
df.filter(pl.col('Age') > 40)
The API is significantly extra complicated than Pandas, but it surely’s useful when you require quick execution for giant datasets. However, you wouldn’t get the profit if the information measurement is small.
To know the small print, you may check with Josep Ferrer’s article on how totally different Polars is are in comparison with Pandas.
4. Vaex
Vaex is much like Polars because the library is developed particularly for appreciable dataset knowledge manipulation. Nevertheless, there are variations in the best way they course of the dataset. For instance, Vaex make the most of memory-mapping strategies, whereas Polars give attention to a multi-threaded strategy.
Vaex is optimally appropriate for datasets which might be manner greater than what Polars meant to make use of. Whereas Polars can also be for in depth dataset manipulation processing, the library is ideally on datasets that also match into reminiscence measurement. On the similar time, Vaex could be nice to make use of on datasets that exceed the reminiscence.
For the Vaex set up, it’s higher to check with their documentation, because it might break your system if it’s not performed appropriately.
5. CuPy
CuPy is an open-source library that permits GPU-accelerated computing in Python. It’s CuPy that was designed for the NumPy and SciPy substitute if you might want to run the calculation inside NVIDIA CUDA or AMD ROCm platforms.
This makes CuPy nice for functions that require intense numerical computation and want to make use of GPU acceleration. CuPy might make the most of the parallel structure of GPU and is helpful for large-scale computations.
To put in CuPy, check with their GitHub repository, as many out there variations would possibly or won’t go well with the platforms you employ. For instance, beneath is for the CUDA platform.
The APIs are much like NumPy, so you need to use CuPy immediately in case you are already conversant in NumPy. For instance, the code instance for CuPy calculation is beneath.
import cupy as cp
x = cp.arange(10)
y = cp.array([2] * 10)
z = x * y
print(cp.asnumpy(z))
CuPy is the top of a necessary Python library in case you are repeatedly working with high-scale computational knowledge.
Conclusion
All of the Python libraries we’ve explored are important in sure use instances. NumPy and Pandas may be the fundamentals, however libraries like Polars, Vaex, and CuPy could be useful in particular environments.
You probably have some other library you deem important, please share them within the feedback!
Cornellius Yudha Wijaya is an information science assistant supervisor and knowledge author. Whereas working full-time at Allianz Indonesia, he likes to share Python and knowledge suggestions by way of social media and writing media. Cornellius writes on a wide range of AI and machine studying matters.
[ad_2]