[ad_1]
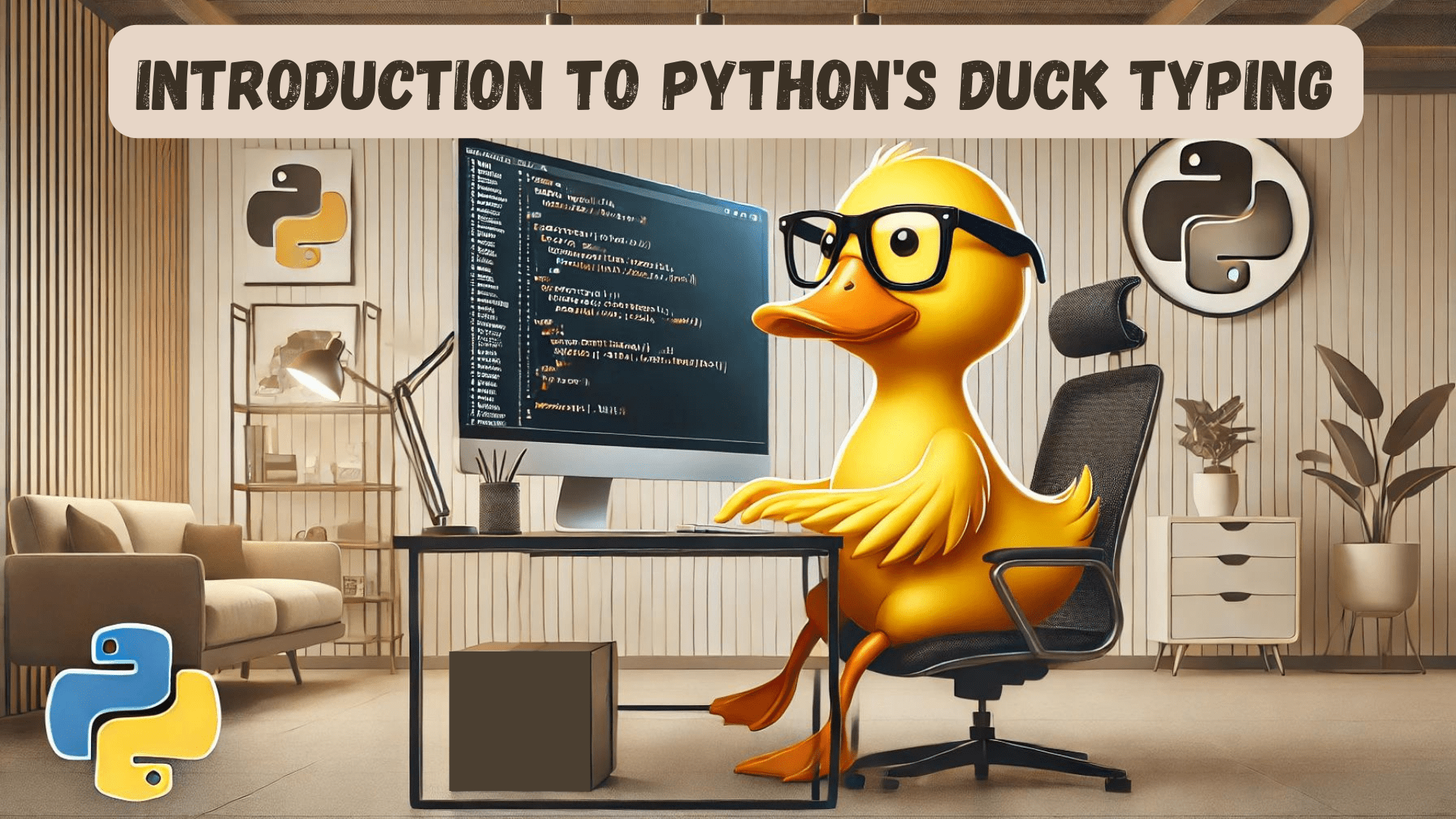
Picture by Writer | DALLE-3 & Canva
What’s Duck Typing?
Duck typing is an idea in programming typically associated to dynamic languages like Python, that emphasizes extra on the article’s habits over its kind or class. Once you use duck typing, you verify whether or not an object has sure strategies or attributes, somewhat than checking for the precise class. The identify comes from the saying,
If it seems to be like a duck, swims like a duck, and quacks like a duck, then it most likely is a duck.
Duck typing brings a number of benefits to programming in Python. It permits for extra versatile and reusable code and helps polymorphism, enabling completely different object varieties for use interchangeably so long as they supply the required interface. This ends in less complicated and extra concise code. Nonetheless, duck typing additionally has its disadvantages. One main disadvantage is the potential for runtime errors. Moreover, it might probably make your code difficult to grasp.
Understanding Dynamic Conduct in Python
In dynamically typed languages, variable varieties should not fastened. As an alternative, they’re decided at runtime primarily based on the assigned values. In distinction, statically typed languages verify variable varieties at compile time. As an illustration, if you happen to try to reassign a variable to a worth of a special kind in static typing, you’ll encounter an error. Dynamic typing offers higher flexibility in how variables and objects are used.
Let’s take into account the *
Python operator; it behaves otherwise primarily based on the kind of the article it’s used with. When used between two integers, it performs multiplication.
# Multiplying two integers
a = 5 * 3
print(a) # Outputs: 15
When used with a string and an integer, it repeats the string. This demonstrates Python’s dynamic typing system and adaptable nature.
# Repeating a string
a="A" * 3
print(a) # Outputs: AAA
How Duck Typing Works in Python?
Duck typing is most well-liked in dynamic languages as a result of it encourages a extra pure coding model. Builders can give attention to designing interfaces primarily based on what objects can do. In duck typing, strategies outlined inside the category are given extra significance than the article itself. Let’s make clear this with a fundamental instance.
Instance No: 01
We have now two lessons: Duck and Particular person. Geese could make a quack sound, whereas individuals can communicate. Every class has a way referred to as sound that prints their respective sounds. The operate make_it_sound
takes any object that has a sound technique and calls it.
class Duck:
def sound(self):
print("Quack!")
class Particular person:
def sound(self):
print("I am quacking like a duck!")
def make_it_sound(obj):
obj.sound()
Now, let’s have a look at how we will use duck typing to work for this instance.
# Utilizing the Duck class
d = Duck()
make_it_sound(d) # Output: Quack!
# Utilizing the Particular person class
p = Particular person()
make_it_sound(p) # Output: I am quacking like a duck!
On this instance, each Duck
and Particular person
lessons have a sound
technique. It does not matter if the article is a Duck or a Particular person; so long as it has a sound
technique, the make_it_sound
operate will work accurately.
Nonetheless, duck typing can result in runtime errors. As an illustration, altering the identify of the tactic sound
within the class Particular person to talk will increase an AttributeError
on runtime. It’s because the operate make_it_sound
expects all of the objects to have a sound operate.
class Duck:
def sound(self):
print("Quack!")
class Particular person:
def communicate(self):
print("I am quacking like a duck!")
def make_it_sound(obj):
obj.sound()
# Utilizing the Duck class
d = Duck()
make_it_sound(d)
# Utilizing the Particular person class
p = Particular person()
make_it_sound(p)
Output:
AttributeError: 'Particular person' object has no attribute 'sound'
Instance No: 02
Let’s discover one other program that offers with calculating areas of various shapes with out worrying about their particular varieties. Every form (Rectangle, Circle, Triangle) has its personal class with a way referred to as space to calculate its space.
class Rectangle:
def __init__(self, width, top):
self.width = width
self.top = top
self.identify = "Rectangle"
def space(self):
return self.width * self.top
class Circle:
def __init__(self, radius):
self.radius = radius
self.identify = "Circle"
def space(self):
return 3.14 * self.radius * self.radius
def circumference(self):
return 2 * 3.14 * self.radius
class Triangle:
def __init__(self, base, top):
self.base = base
self.top = top
self.identify = "Triangle"
def space(self):
return 0.5 * self.base * self.top
def print_areas(shapes):
for form in shapes:
print(f"Space of {form.identify}: {form.space()}")
if hasattr(form, 'circumference'):
print(f"Circumference of the {form.identify}: {form.circumference()}")
# Utilization
shapes = [
Rectangle(4, 5),
Circle(3),
Triangle(6, 8)
]
print("Areas of various shapes:")
print_areas(shapes)
Output:
Areas of various shapes:
Space of Rectangle: 20
Space of Circle: 28.259999999999998
Circumference of the Circle: 18.84
Space of Triangle: 24.0
Within the above instance, we’ve got a print_areas
operate that takes an inventory of shapes and prints their names together with their calculated areas. Discover that we need not verify the kind of every form explicitly earlier than calculating its space. As the tactic circumference
is barely current for the Circle
class, it will get carried out solely as soon as. This instance exhibits how duck typing can be utilized to jot down versatile code.
Closing Ideas
Duck typing is a strong function of Python that makes your code extra dynamic and versatile, enabling you to jot down extra generic and adaptable packages. Whereas it brings many advantages, reminiscent of flexibility and ease, it additionally requires cautious documentation and testing to keep away from potential errors.
Kanwal Mehreen Kanwal is a machine studying engineer and a technical author with a profound ardour for knowledge science and the intersection of AI with medication. She co-authored the e-book “Maximizing Productiveness with ChatGPT”. As a Google Technology Scholar 2022 for APAC, she champions range and tutorial excellence. She’s additionally acknowledged as a Teradata Variety in Tech Scholar, Mitacs Globalink Analysis Scholar, and Harvard WeCode Scholar. Kanwal is an ardent advocate for change, having based FEMCodes to empower girls in STEM fields.
[ad_2]