With regards to working your AR course of on NetSuite, probably the most widespread duties you will do is creating gross sales orders.
Manually creating gross sales orders might be tedious – the NetSuite UI just isn’t very easy, and there are additional complexities which are current solely within the API and never on the NetSuite UI utility.
Nevertheless, the complexity of the NetSuite API might be tough to take care of. It takes numerous devoted time (and energy) to arrange an API integration and really automate your NetSuite workflows.
On this information we’ll undergo a step-by-step course of on the way to use the NetSuite API to create gross sales orders, in addition to automation options that may make the job simpler.
Nanonets automates gross sales order entry into NetSuite, and units up seamless 2-way matching with funds in lower than quarter-hour!
Understanding Gross sales Orders in NetSuite
A Gross sales Order is a transaction between a enterprise and a buyer the place the client commits to buying services or products. Gross sales orders are essential for monitoring stock, income, and buyer funds. NetSuite’s API permits you to create, handle, and fulfill these orders programmatically.
There are 4 predominant kinds of gross sales orders in NetSuite:
- Customary Gross sales Order: The default sort for normal gross sales transactions the place fee is due upon supply.
- Customary Gross sales Order – Money: Used for gross sales transactions the place fee is made on the time of the sale.
- Customary Gross sales Order – Bill: Permits the sale to be invoiced at a later time, typically after the services or products has been delivered.
- Customary Gross sales Order – Progress Billing: Used for giant initiatives that require billing in phases or milestones, as work progresses.
The usual gross sales order is essentially the most versatile of all of those, and it gives you essentially the most variety of choices to enter as fields. If you create a regular gross sales order, you mainly want to decide on these 5-6 fields:
- The client
- The transaction date (defaulted to the present date)
- The gross sales order standing (Pending Approval or Pending Achievement)
- The gadgets/companies that you’re promoting
- The fee phrases
- The fee technique
Nevertheless, in lots of instances, it is perhaps useful to make use of one of many different 3 kinds of gross sales orders – particularly once you already know prematurely what sort of sale it’s going to be (for eg. a money sale). The profit to doing that’s that most of the fields shall be pre-populated for you, saving effort and time.
Establishing the NetSuite API
Earlier than you can begin creating gross sales orders utilizing the NetSuite API, you will must arrange your account entry and guarantee correct authentication.
We now have printed a separate, detailed information on establishing the NetSuite API – you may all the time get began there after which come again right here.
In case you already know the way to run API calls and simply want quick-start directions for NetSuite authentication, right here’s how you are able to do it:
Get hold of Account Credentials:
- Log in to your NetSuite account.
- Navigate to Setup > Firm > Allow Options.
- Below the SuiteCloud tab, make sure that REST Internet Companies and Token-Based mostly Authentication are enabled.
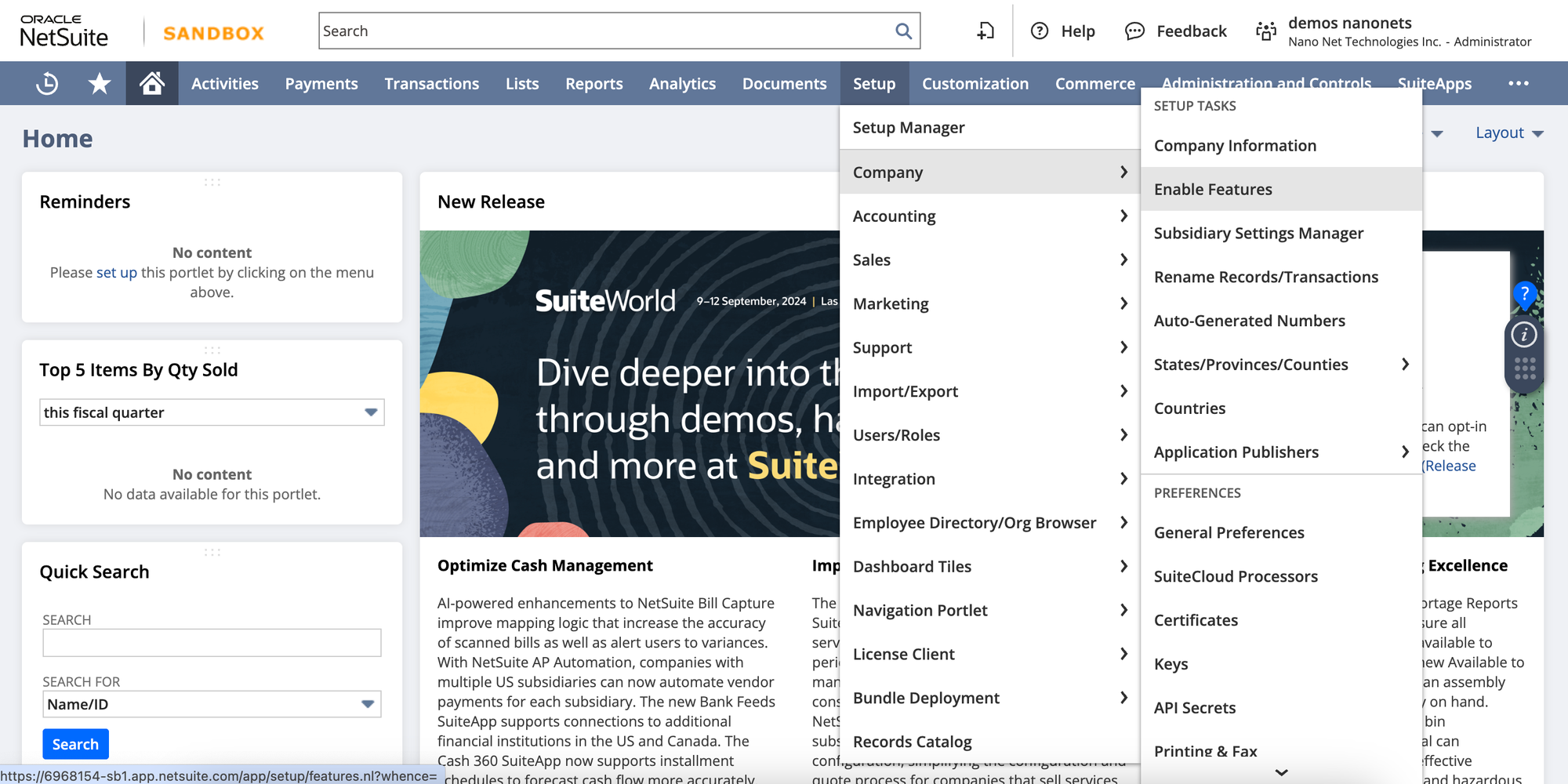
Create an Integration Report:
- Go to Setup > Integration > Handle Integrations > New.
- Fill out the required fields, and observe down the Shopper Key and Shopper Secret supplied.
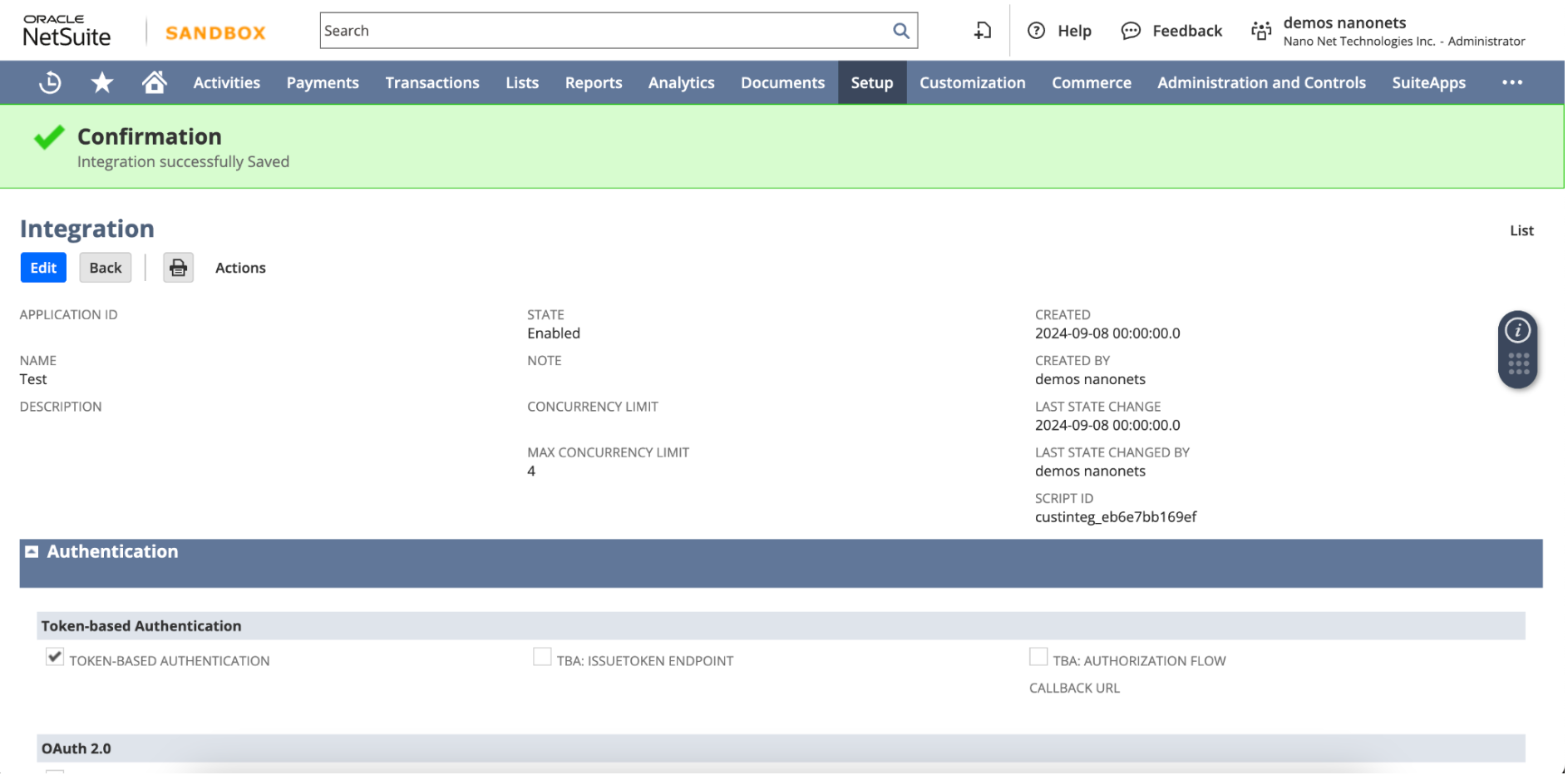
Set Up Token-Based mostly Authentication (TBA):
- Navigate to Setup > Customers/Roles > Entry Tokens > New.
- Choose the mixing report you created, and generate the Token ID and Token Secret.
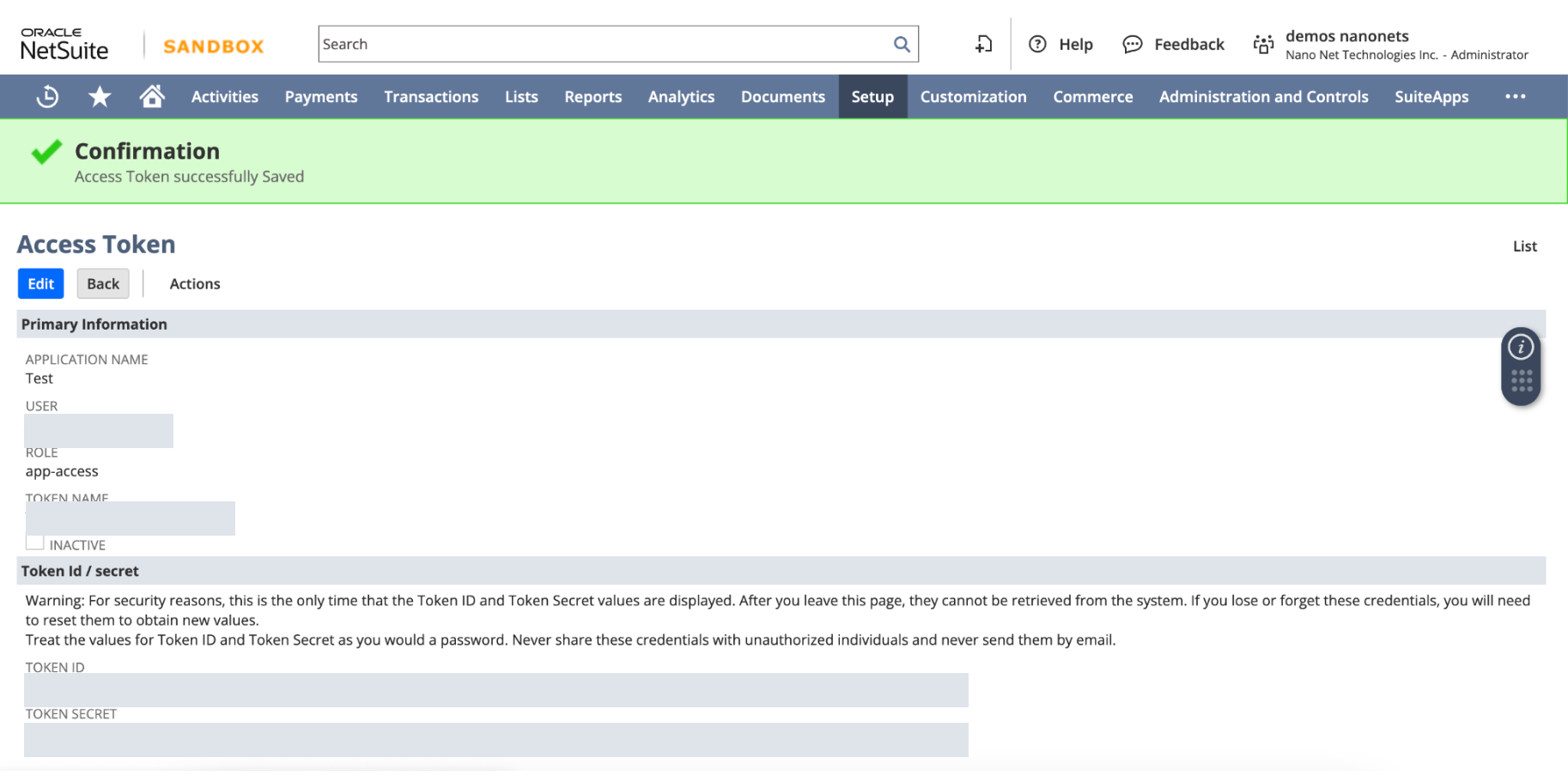
Together with your Account ID, Shopper Key, Shopper Secret, Token ID, and Token Secret, you are now able to make authenticated API calls to NetSuite.
Making a Gross sales Order in NetSuite
The NetSuite API permits you to create Gross sales Orders programmatically, guaranteeing seamless integration between techniques. Beneath is a step-by-step information to making a Gross sales Order utilizing NetSuite’s REST API in python.
Authentication
Earlier than making any API calls to NetSuite, it’s essential authenticate utilizing OAuth 1.0. The next Python code makes use of the requests_oauthlib
library to authenticate the request utilizing your consumer_key
, consumer_secret
, token_key
, and token_secret
.
This is the way you authenticate utilizing OAuth 1.0:
import requests
from requests_oauthlib import OAuth1
# Authentication particulars
auth = OAuth1('consumer_key', 'consumer_secret', 'token_key', 'token_secret')
When you’re authenticated, you are able to construct your payloads.
Create Gross sales Order Payload
That is the primary payload that sends the gross sales order knowledge to NetSuite. On this, we’re in a position to outline what sort of gross sales order we’re creating. We take 2 examples beneath from the 4 kinds of gross sales orders that we noticed earlier.
1. Customary Merchandise-Based mostly Gross sales Order
In case your gross sales order entails tangible merchandise, right here’s the way to construction the payload for the standard gross sales order:
item_payload = {
"entity": {"id": "1234"}, # Buyer ID
"trandate": "2024-09-01", # Transaction Date
"duedate": "2024-09-15", # Due Date
"foreign money": {"id": "1"}, # Forex ID (USD)
"phrases": {"id": "1"}, # Fee phrases
"merchandise": [
{
"item": {"id": "5678"}, # Item ID
"quantity": 10, # Quantity
"rate": 20.00 # Unit price
}
],
"memo": "Gross sales order for workplace provides"
}
2. Progress Billing Gross sales Order
For big initiatives which are billed incrementally, right here’s the construction for a progress billing gross sales order:
progress_billing_payload = {
"entity": {"id": "5678"}, # Buyer ID
"trandate": "2024-09-01", # Transaction Date
"duedate": "2024-09-15", # Due Date
"foreign money": {"id": "1"}, # Forex ID (USD)
"merchandise": [
{
"item": {"id": "service_item_id"}, # Service Item ID
"quantity": 1, # Quantity
"rate": 200.00, # Rate
"percentcomplete": 50 # Percentage complete for progress billing
}
],
"memo": "Consulting companies for September"
}
Ship POST Request
As soon as the payload is created, the subsequent step is to ship a POST request to the NetSuite API utilizing the authenticated session. Beneath is the operate to deal with the request:
def create_sales_order(auth, payload):
url = "https://<account_id>.suitetalk.api.netsuite.com/companies/relaxation/report/v1/salesOrder"
headers = {"Content material-Kind": "utility/json"}
# Ship POST request to create the gross sales order
response = requests.put up(url, json=payload, headers=headers, auth=auth)
return response
# Ship the gross sales order creation request
response = create_sales_order(auth, payload)
URL: That is the NetSuite REST API endpoint for creating gross sales orders. Change <account_id>
together with your precise NetSuite account ID.
Response Dealing with
As soon as the POST request is made, the API returns a response. It is best to examine the standing of the response and deal with it accordingly. If the gross sales order is created efficiently, the response will embrace particulars such because the gross sales order ID and standing.
# Response Dealing with
if response.status_code == 200:
print("Gross sales Order created efficiently:", response.json())
else:
print("Error creating Gross sales Order:", response.status_code, response.textual content)
Instance of profitable Gross sales Order creation
As soon as the gross sales order is created efficiently it would present up within the NetSuite UI as beneath:
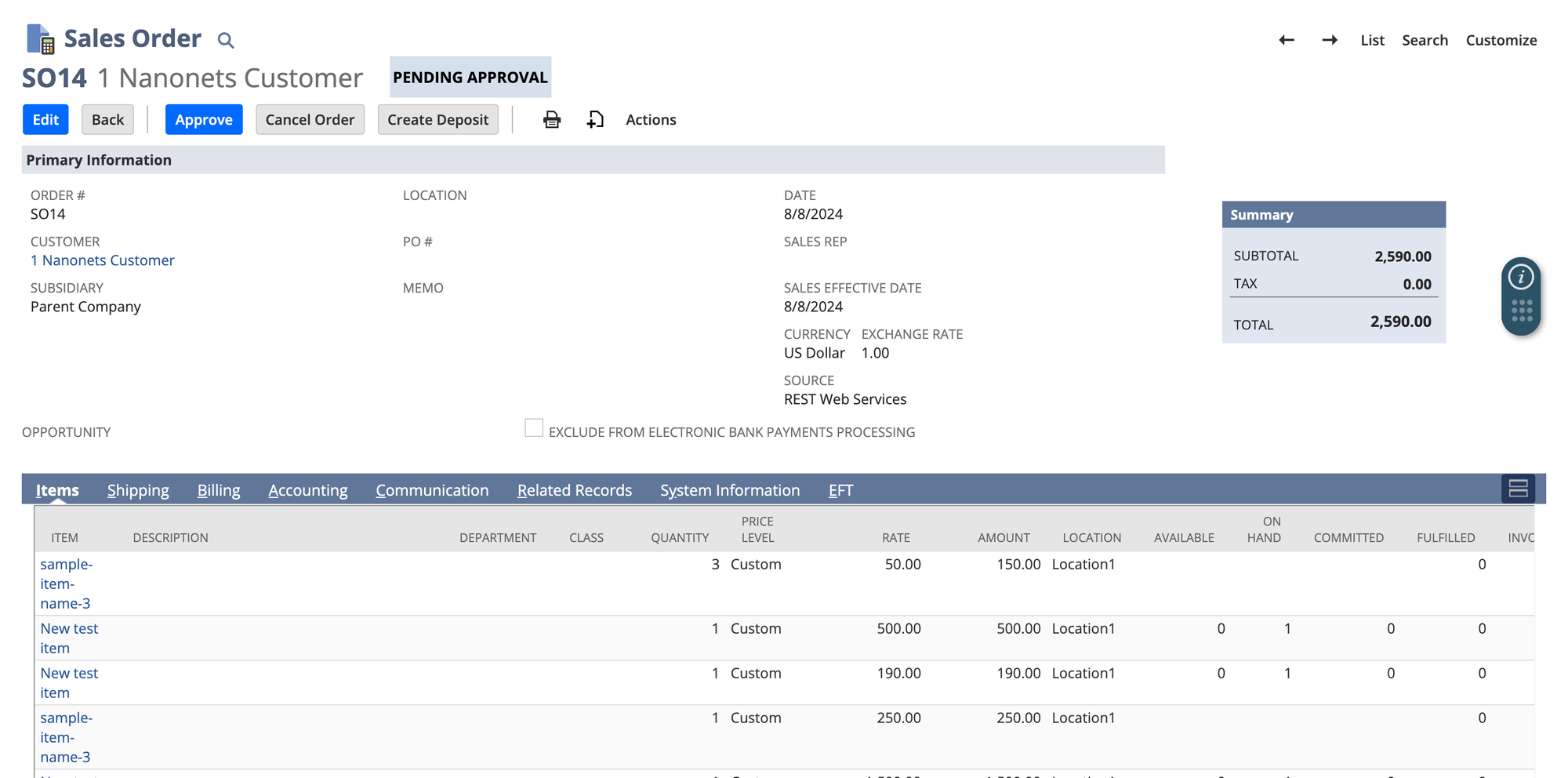
Obtain Full Code:
The core code for posting a gross sales order utilizing NetSuite stays constant. Nevertheless, the payload varies relying on the kind of gross sales order being added. Let’s discover the way to ship a POST request so as to add varied kinds of gross sales orders utilizing the NetSuite API.
Easy methods to Retrieve Inner IDs in NetSuite
To populate the required fields within the payloads (reminiscent of itemID
, currencyID
, termsID
, and accountID
), you will want to make use of NetSuite’s Inner IDs. These IDs correspond to particular entities, gadgets, or phrases inside your NetSuite account.
You’ll find these inside IDs by way of the next steps:
- NetSuite UI: Navigate to the related report (e.g., Vendor, Merchandise, or Account) within the NetSuite dashboard and allow “Inner IDs” below
Residence -> Set Preferences -> Common -> Defaults -> Present Inner IDs
.
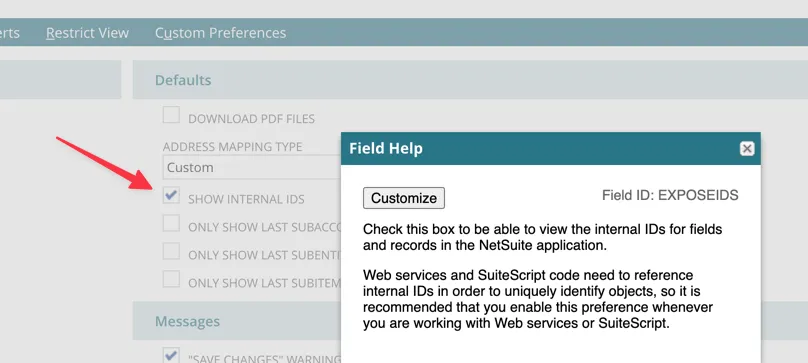
- Saved Searches: Navigate to Studies -> Saved Searches -> All Saved Searches within the NetSuite dashboard. Create a brand new search and choose the related report sort (e.g., Objects, Clients). Within the Outcomes tab, add the Inner ID area as a column. Working the search will show the inner IDs of the chosen information in your outcomes.
- SuiteScript: You may write and deploy a SuiteScript that retrieves inside IDs from information. After deploying the script, you may run it to entry inside IDs to be used in your API calls. Beneath an instance of a easy SuiteScript to retrieve inside IDs of consumers:
/**
* @NApiVersion 2.x
* @NScriptType Suitelet
*/
outline(['N/search'], operate(search) {
operate onRequest(context) {
var customerSearch = search.create({
sort: search.Kind.CUSTOMER,
columns: ['internalid', 'entityid'] // inside ID and Buyer title
});
var searchResult = customerSearch.run();
var prospects = [];
searchResult.every(operate(outcome) {
prospects.push({
internalId: outcome.getValue({ title: 'internalid' }),
customerName: outcome.getValue({ title: 'entityid' })
});
return true; // proceed iteration
});
// Ship again response as JSON
context.response.write(JSON.stringify(prospects));
}
return {
onRequest: onRequest
};
});
With these inside IDs, you may guarantee your API calls goal the right information, enhancing accuracy and effectivity in your gross sales order creation course of.
Dealing with Gross sales Order Fulfilment and Fee Situations in NetSuite
When creating gross sales orders in NetSuite, the kind of fee phrases that you simply’re making use of can differ, and can typically rely upon one other variable – when the gross sales order is getting fulfilled.
There are just a few widespread duties that you’ll often must do:
- Apply a buyer fee to a Gross sales Order
- Dealing with reductions and credit
- Remodel a Gross sales Order into an Merchandise Fulfilment
Making use of Buyer Funds to Gross sales Orders
As soon as a gross sales order is created, buyer funds might be utilized utilizing this payload:
payment_data = {
"buyer": {"id": "customer_id"},
"salesOrder": {"id": "sales_order_id"},
"fee": {
"quantity": 500,
"technique": {"id": "payment_method_id"} # Fee Methodology ID (e.g., bank card)
}
}
payment_url="https://your_netsuite_instance/relaxation/customerpayment/v1/"
response = requests.put up(payment_url, json=payment_data, auth=auth)
print(response.json())
Making use of Reductions or Credit to Gross sales Orders
To use reductions or credit to a gross sales order, replace the payload like this:
sales_order_data["discountitem"] = {"id": "discount_id"} # Low cost ID
response = requests.put up(url, json=sales_order_data, auth=auth)
print(response.json())
Remodel a Gross sales Order into an Merchandise Fulfilment
As soon as a gross sales order is able to be fulfilled, you may rework the gross sales order into an merchandise success utilizing the NetSuite API. This course of ensures that the gadgets within the gross sales order are marked as shipped or delivered.
This may be executed as a NetSuite rework – i.e., you modify the gross sales order to an merchandise fulfilment.
Right here’s an instance API name for instantly reworking a gross sales order into merchandise success:
POST /report/v1/salesOrder/<Sales_Order_id>/!rework/itemFulfillment
{
"inventoryLocation": {
"id" : <location id>
}
...
}
Be aware that the inventoryLocation area is obligatory when reworking a Gross sales Order to an Merchandise Achievement (it’s essential specify from the place within the stock you’re delivery the gadgets that you simply simply offered).
Frequent Pitfalls and Troubleshooting
Creating gross sales orders through the NetSuite API is a robust solution to automate your AR course of, however it comes with its challenges. Listed below are some widespread pitfalls and the way to keep away from them:
Pitfall | Answer |
---|---|
Authentication Points | Double-check your tokens, keys, and permissions. Make sure that Token-Based mostly Authentication (TBA) is appropriately arrange. |
Lacking or Incorrect Fields | At all times seek advice from the NetSuite API documentation to make sure all required fields are included and appropriately formatted. |
Information Synchronization Points | Implement common GET queries to confirm that the info in your system matches what’s in NetSuite. Think about using middleware or an integration platform to keep up synchronization. |
Fee Limits | Monitor your API utilization and implement methods like request batching or throttling to remain inside limits. |
Dealing with Partial Exports | Implement error dealing with and logging to establish and tackle partial exports promptly. |
Create Gross sales Orders on Netsuite utilizing Nanonets
Nanonets simplifies the method by dealing with the complexities of API authentication, scope administration, and error dealing with, making it an perfect end-to-end NetSuite automation workflow supplier.
By leveraging the AI-powered Nanonets platform, you may automate the complete vendor invoice creation course of in NetSuite with minimal human intervention. This ensures quicker processing occasions, improved accuracy, and seamless integration together with your current workflows.
Nanonets intelligently extracts key knowledge out of your inside CRM in addition to paperwork, maps it instantly into NetSuite, and generates correct gross sales orders.
Right here’s a demo video displaying how one can arrange automated NetSuite workflows utilizing Nanonets in below 2 minutes.
Benefits of Utilizing Nanonets:
- Native NetSuite Connection: Handles all of the intricate particulars like API authentication and lacking fields.
- Out-of-the-Field Workflows: Simplifies duties like gross sales order creation and fee utility.
- Correct Information Extraction: Leverages Gen AI fashions to extract knowledge from invoices and different paperwork.
- Consumer and Information Administration: Offers options like doc storage and administration and workforce collaboration.
- Approval Workflows: Permits for knowledge verification inside your workforce earlier than posting to NetSuite.
By automating the gross sales order creation course of with Nanonets, you may guarantee effectivity, accuracy, and scalability in your accounts receivable workflow, leaving the tedious duties to AI.
For extra data, try the Nanonets documentation.
Nanonets automates gross sales order and fee entry into NetSuite, plus units up seamless 2-way, 3-way, and 4-way matching in minutes!
Conclusion
Creating gross sales orders in NetSuite utilizing the API streamlines your accounts receivable course of, from dealing with a number of gadgets and recurring funds to making use of reductions.
However why cease at simply handbook API calls? Nanonets takes automation a step additional. By harnessing AI, Nanonets automates the extraction and enter of essential gross sales order knowledge instantly into NetSuite, saving you time and eliminating the danger of human error.